#include<stdio.h>
#include<stdlib.h>
//prototypes of used function
void push();
void pop();
void display();
/*structure of stack node */
typedef struct node
{
int data;
struct node *next;
} sNode;
sNode *top = NULL;
/*driver function to test the stack operations */
int main(int argc, char * argv[])
{
int choice ;
do{
start :
choice =0; /* it will take care of if user entered any invalid choice */
printf("\nValid Stack Operations \n1. push an item\n2. pop an item \n3. display stack\n0. Exit\n");
printf("+-------------------------------+\n");
printf("Enter your choice : ");
scanf("%d", &choice); /* Here if your input is invalid program will be terminated simply without crashing */
switch(choice)
{
case 1: push();
break;
case 2: pop();
break;
case 3: display();
break;
case 0: exit(1);
break;
default :
printf("\nYou have entered wrong choice, try again !\n");
goto start;
}
}while(choice != 0);
return 0;
}
/*function to display the stack status */
void display()
{
sNode * temp;
temp = top;
printf("\n top -->\n");
if(temp == NULL)
printf("\t|_______|\n\t+-Stack-+\n Stack is Empty !\n") ;
while(temp != NULL)
{
printf("\t| %d\t|\n",temp->data);
temp = temp->next;
}
/* it is just for visuality nothing else */
if(top != NULL)
printf("\t+-------+\n\t++Stack++\n");
}
/* function to insert the data in to stack */
void push()
{
int num;
sNode *temp;
printf("Enter the data : ");
scanf("%d", &num);
/* allocating memory to new stack node*/
temp = (sNode *) malloc (sizeof(sNode));
temp->data = num;
temp->next = NULL;
/* insert the data at top of stack*/
if(top == NULL){
top = temp;
}
else{
temp->next = top;
top = temp;
}
printf("\ndata successfully inserted to stack !\n");
}
/*function to delete the data from stack */
void pop()
{
sNode * temp;
temp = top;
if(temp != NULL){ /*check whether Stack is Empty or not */
top = top->next;
free(temp);
printf("\npop operation successfully completed !\n");
}
else
printf("\nStack is Empty you can't perform pop operation !\n");
}
Output: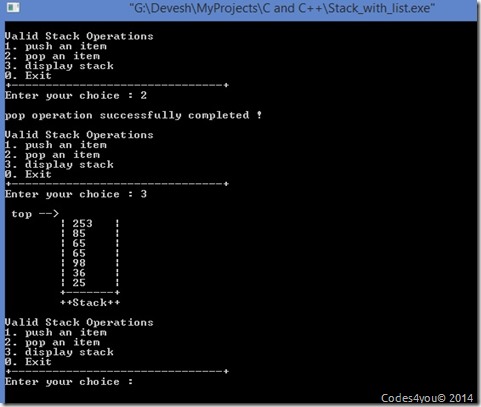